Golang中的加密与安全实践
Golang 中的加密与安全实践
Golang 是一种功能强大的编程语言,被广泛用于开发各种类型的应用程序。由于其高效性和可靠性,Golang 也开始在安全领域得到广泛应用。在本文中,我们将深入探讨 Golang 中的加密与安全实践。
1. 密码学
密码学是一种用于保护数据和通信的数学科学。Golang 中的密码学是基于标准库 crypto 的。
1.1 对称加密
对称加密是一种加密技术,使用相同的密钥进行加密和解密。Golang 中支持的对称加密算法包括:DES、3DES、AES、Blowfish、RC4 等。其中 AES 是最常用的。以下代码展示了如何使用 AES 对称加密和解密数据:
`go
package main
import (
"crypto/aes"
"crypto/cipher"
"crypto/rand"
"fmt"
"io"
)
func main() {
key := byte("0123456789abcdef")
plaintext := byte("Hello, world!")
nonce := make(byte, 12)
if _, err := io.ReadFull(rand.Reader, nonce); err != nil {
panic(err)
}
block, err := aes.NewCipher(key)
if err != nil {
panic(err)
}
aesgcm, err := cipher.NewGCM(block)
if err != nil {
panic(err)
}
ciphertext := aesgcm.Seal(nil, nonce, plaintext, nil)
fmt.Printf("%x\n", ciphertext)
plaintext2, err := aesgcm.Open(nil, nonce, ciphertext, nil)
if err != nil {
panic(err)
}
fmt.Printf("%s\n", plaintext2)
}
1.2 非对称加密非对称加密使用公钥和私钥两个不同的密钥进行加密和解密。Golang 中支持的非对称加密算法包括:RSA、DSA、ECDSA 等。以下代码展示了如何使用 RSA 非对称加密和解密数据:`gopackage mainimport ( "crypto/rand" "crypto/rsa" "crypto/x509" "encoding/pem" "fmt")func main() { plaintext := byte("Hello, world!") privkey, err := rsa.GenerateKey(rand.Reader, 2048) if err != nil { panic(err) } pubkey := privkey.PublicKey ciphertext, err := rsa.EncryptPKCS1v15(rand.Reader, &pubkey, plaintext) if err != nil { panic(err) } plaintext2, err := rsa.DecryptPKCS1v15(rand.Reader, privkey, ciphertext) if err != nil { panic(err) } fmt.Printf("%s\n", plaintext2)}
2. 安全
2.1 密码处理
密码处理是非常重要的安全实践。Golang 中的密码处理可以通过 bcrypt 等散列函数来实现。以下代码展示了如何使用 bcrypt 生成和验证密码哈希值:
`go
package main
import (
"fmt"
"golang.org/x/crypto/bcrypt"
)
func main() {
password := byte("mypassword")
// 加密密码
hash, err := bcrypt.GenerateFromPassword(password, bcrypt.MinCost)
if err != nil {
panic(err)
}
fmt.Printf("%x\n", hash)
// 验证密码
err = bcrypt.CompareHashAndPassword(hash, password)
if err != nil {
panic(err)
}
fmt.Println("Password validated")
}
2.2 TLSTLS(Transport Layer Security)是一种用于保护通信的协议,其前身是 SSL(Secure Sockets Layer)。Golang 中的 TLS 可以通过标准库 crypto/tls 来实现。以下代码展示了如何使用 TLS 连接到 HTTP 服务器:`gopackage mainimport ( "crypto/tls" "fmt" "net/http")func main() { tr := &http.Transport{ TLSClientConfig: &tls.Config{InsecureSkipVerify: true}, } client := &http.Client{Transport: tr} resp, err := client.Get("https://www.example.com") if err != nil { panic(err) } defer resp.Body.Close() fmt.Println(resp.StatusCode)}
总结
在本文中,我们介绍了 Golang 中的加密与安全实践。我们讨论了密码学、密码处理和 TLS 三个方面的内容。这些技术可以使您的应用程序更加安全,并保护您的用户数据不被攻击者窃取。我们希望这篇文章能够帮助您更好地理解 Golang 中的安全性和加密实践。

猜你喜欢LIKE
相关推荐HOT
更多>>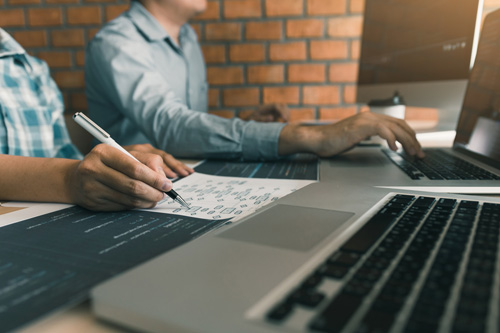
利用Golang构建分布式系统
利用Golang构建分布式系统——如何利用Golang实现高效的分布式系统分布式系统是一种采用多个计算机节点协同工作的系统,该系统的目标是提供一个...详情>>
2023-12-24 22:38:57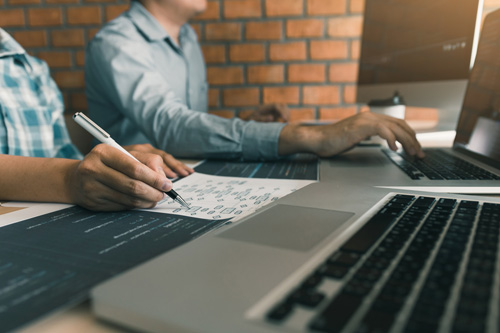
如何保障你的云计算服务的可用性
如何保障你的云计算服务的可用性云计算服务已成为现代企业的标配,其无需维护硬件,按需使用的模式大大降低了IT部门的成本。但是,云计算服务也...详情>>
2023-12-24 17:50:57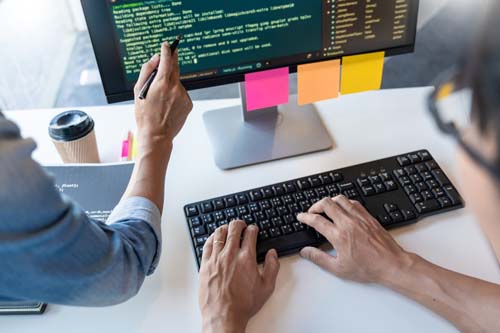
如何优化MySQL数据库的性能,提升应用响应速度
MySQL是一款非常常用的关系型数据库管理系统,用于存储和管理大量数据。在高并发的应用场景下,优化MySQL数据库的性能成为了一项必须要做的工作...详情>>
2023-12-24 15:26:57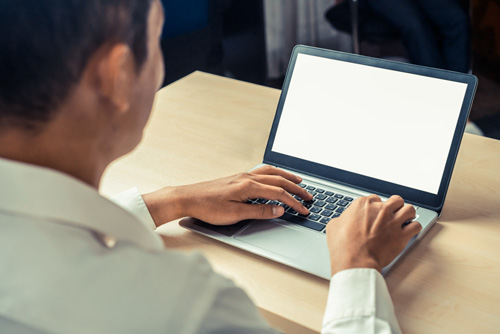
最佳实践如何在Kubernetes中部署应用程序
最佳实践:如何在Kubernetes中部署应用程序在现代化的云操作系统环境中,Kubernetes已经成为了企业级应用程序的首选平台。Kubernetes提供了一种...详情>>
2023-12-24 07:02:56热门推荐
Golang中的加密与安全实践
沸利用Golang构建分布式系统
热Goland必知必会的三个技巧
热如何构建一个云原生的应用程序?
新五个常用的Docker应用场景
如何保障你的云计算服务的可用性
如何利用Docker容器实现快速部署和管理应用?
如何优化MySQL数据库的性能,提升应用响应速度
如何使用Nginx和Apache部署Web服务器
OpenStack架构详解,掌握云计算的核心技术
如何使用Ansible在Linux上自动化部署?
如何在AWS上利用Lambda函数实现自动化任务
Docker在云计算中的应用为什么越来越受欢迎?
云计算时代的存储架构为什么你需要考虑使用对象存储